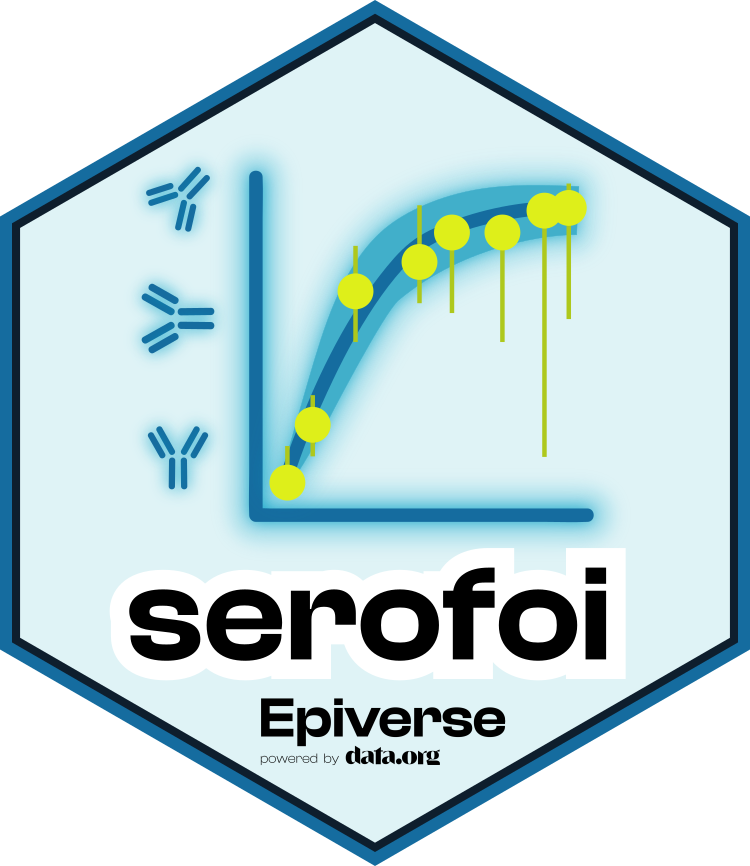
Simulate serosurvey data based on general serocatalytic model.
simulate_serosurvey_general_model.Rd
This simulation method assumes only that the model system can be written as a piecewise-linear ordinary differential equation system.
Usage
simulate_serosurvey_general_model(
construct_A_function,
calculate_seropositivity_function,
initial_conditions,
survey_features,
...
)
Arguments
- calculate_seropositivity_function
A function which takes the state vector and returns the seropositive fraction.
- initial_conditions
The initial state vector proportions for each birth cohort.
- survey_features
A dataframe containing information about the binned age groups and sample sizes for each. It should contain columns: 'age_min', 'age_max', 'sample_size'.