Tracking Changes
Last updated on 2024-03-12 | Edit this page
Estimated time: 45 minutes
Overview
Questions
- How do I record changes in Git?
- How do I check the status of my version control repository?
- How do I record notes about what changes I made and why?
Objectives
- Go through the modify-add-commit cycle for one or more files.
- Explain where information is stored at each stage of that cycle.
- Distinguish between descriptive and non-descriptive commit messages.
Let’s start to tell the story of your project while you are working on it!
Add changes
First, in the Rstudio Console, let’s make sure we’re
still in the right R project. You should be in the cases
directory.
R
usethis::proj_path()
OUTPUT
C:/~/cases
Let’s create a file called sitrep.Rmd
that contains the
situation report (Sitrep) describing the data in terms of person, time, and
place.
We can do this from the Console. Run:
R
usethis::edit_file("sitrep.Rmd")
• Modify 'sitrep.Rmd'
This opens the file in the Source pane to edit it.
Type the text below into the sitrep.Rmd
file:
OUTPUT
Comparison of attack rates in different age groups
Save the file.
Checklist
Remember always to Save the file. Only saved files are recognized by Git.
Now, in the Terminal, if we check the status of our project again, Git tells us that it’s noticed the new file:
OUTPUT
On branch main
No commits yet
Untracked files:
(use "git add <file>..." to include in what will be committed)
sitrep.Rmd
nothing added to commit but untracked files present (use "git add" to track)
The “untracked files” message means that there’s a file in the
directory that Git isn’t keeping track of. We can tell Git to track a
file using git add
:
and then check that the right thing happened:
OUTPUT
On branch main
No commits yet
Changes to be committed:
(use "git rm --cached <file>..." to unstage)
new file: sitrep.Rmd
Commit changes
Git now knows that it’s supposed to keep track of
sitrep.Rmd
, but it hasn’t recorded these changes as a
commit yet. To get it to do that, in the Terminal, we
need to run one more command:
OUTPUT
[main (root-commit) f22b25e] Start Sitrep with attack rate analysis
1 file changed, 1 insertion(+)
create mode 100644 sitrep.Rmd
When we run git commit
, Git takes everything we have
told it to save by using git add
and stores a copy
permanently inside the special .git
directory. This
permanent copy is called a commit
(or revision) and its short
identifier is f22b25e
. Your commit may have another
identifier.
We use the -m
flag (for “message”) to record a short,
descriptive, and specific comment that will help us remember later on
what we did and why. If we just run git commit
without the
-m
option, Git will launch VIM
(or whatever
other editor we configured as core.editor
) so that we can
write a longer message.
Exiting Vim
Note that Vim is the default editor for many programs. If you haven’t
used Vim before and wish to exit a session without saving your changes,
press Esc then type :q!
and hit Enter
or ↵ or on Macs, Return. If you want to save your
changes and quit, press Esc then type :wq
and
hit Enter or ↵ or on Macs, Return.
With Rstudio you don’t need to do change any editor. You can open all your files in the Source pane.
Also, to write commit messages you can use the Rstudio IDE. We invite you to read the supplemental episode.
However, if needed, Dracula can set his favorite text editor following this table:
Editor | Configuration command |
---|---|
Atom | $ git config --global core.editor "atom --wait" |
nano | $ git config --global core.editor "nano -w" |
BBEdit (Mac, with command line tools) | $ git config --global core.editor "bbedit -w" |
Sublime Text (Mac) | $ git config --global core.editor "/Applications/Sublime\ Text.app/Contents/SharedSupport/bin/subl -n -w" |
Sublime Text (Win, 32-bit install) | $ git config --global core.editor "'c:/program files (x86)/sublime text 3/sublime_text.exe' -w" |
Sublime Text (Win, 64-bit install) | $ git config --global core.editor "'c:/program files/sublime text 3/sublime_text.exe' -w" |
Notepad (Win) | $ git config --global core.editor "c:/Windows/System32/notepad.exe" |
Notepad++ (Win, 32-bit install) | $ git config --global core.editor "'c:/program files (x86)/Notepad++/notepad++.exe' -multiInst -notabbar -nosession -noPlugin" |
Notepad++ (Win, 64-bit install) | $ git config --global core.editor "'c:/program files/Notepad++/notepad++.exe' -multiInst -notabbar -nosession -noPlugin" |
Kate (Linux) | $ git config --global core.editor "kate" |
Gedit (Linux) | $ git config --global core.editor "gedit --wait --new-window" |
Scratch (Linux) | $ git config --global core.editor "scratch-text-editor" |
Emacs | $ git config --global core.editor "emacs" |
Vim | $ git config --global core.editor "vim" |
VS Code | $ git config --global core.editor "code --wait" |
It is possible to reconfigure the text editor for Git whenever you want to change it.
Good commit
messages start with a brief (<50 characters) statement about the
changes made in the commit. Generally, the message should complete the
sentence “If applied, this commit will”
Good practice
A good commit:
Contains less than 50 characters in first line.
Start with an infinitive verb.
Recalls an specific action.
If we run git status
now:
OUTPUT
On branch main
nothing to commit, working tree clean
it tells us everything is up to date.
Show the history
If we want to know what we’ve done recently, in the
Terminal, we can ask Git to show us the project’s history using
git log
:
OUTPUT
commit f22b25e3233b4645dabd0d81e651fe074bd8e73b
Author: Vlad Dracula <vlad@tran.sylvan.ia>
Date: Thu Aug 22 09:51:46 2013 -0400
Start Sitrep with attack rate analysis
git log
lists all commits made to a repository in
reverse chronological order. The listing for each commit includes the
commit’s full identifier (which starts with the same characters as the
short identifier printed by the git commit
command
earlier), the commit’s author, when it was created, and the log message
Git was given when the commit was created.
These commands are so similar!
The git status
command displays the state of the
working directory and the staging area. It lets you
see which changes have been staged, which haven’t, and which files
aren’t being tracked by Git. Status output does not show
you any information regarding the committed project history
(the local repository). For this, you need to use
git log
. (Atlassian,
2023)
Where Are My Changes?
If, in the Terminal, we run ls
at this point, we will
still see just one file called sitrep.Rmd
. That’s because
Git saves information about files’ history in the special
.git
directory mentioned earlier so that our filesystem
doesn’t become cluttered (and so that we can’t accidentally edit or
delete an old version).
Compare changes
Now suppose Dracula adds more information to the file. For this,
let’s return to the sitrep.Rmd
file:
R
usethis::edit_file("sitrep.Rmd")
OUTPUT
Comparison of attack rates in different age groups
This can identify priority groups for interventions
Save the file.
In the Terminal, when we run git status
now, it tells us that a file it already knows about has been
modified:
OUTPUT
On branch main
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git checkout -- <file>..." to discard changes in working directory)
modified: sitrep.Rmd
no changes added to commit (use "git add" and/or "git commit -a")
The last line is the key phrase: “no changes added to commit”. We
have changed this file, but we haven’t told Git we will want to save
those changes (which we do with git add
) nor have we saved
them (which we do with git commit
). So let’s do that now.
It is good practice to always review our changes before saving them. In
the Terminal, we do this using git diff
. This shows us the
differences between the current state of the file and the most recently
saved version:
OUTPUT
diff --git a/sitrep.Rmd b/sitrep.Rmd
index df0654a..315bf3a 100644
--- a/sitrep.Rmd
+++ b/sitrep.Rmd
@@ -1 +1,2 @@
Comparison of attack rates in different age groups
+This can identify priority groups for interventions
The output is cryptic because it is actually a series of commands for
tools like editors and patch
telling them how to
reconstruct one file given the other. If we break it down into
pieces:
- The first line tells us that Git is producing output similar to the
Unix
diff
command comparing the old and new versions of the file. - The second line tells exactly which versions of the file Git is
comparing;
df0654a
and315bf3a
are unique computer-generated labels for those versions. - The third and fourth lines once again show the name of the file being changed.
- The remaining lines are the most interesting, they show us the
actual differences and the lines on which they occur. In particular, the
+
marker in the first column shows where we added a line.
Staging area
After reviewing our change, it’s time to commit it:
OUTPUT
On branch main
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git checkout -- <file>..." to discard changes in working directory)
modified: sitrep.Rmd
no changes added to commit (use "git add" and/or "git commit -a")
Whoops: Git won’t commit because we didn’t use git add
first. Let’s fix that:
OUTPUT
[main 34961b1] Add purpose of attack rate analysis
1 file changed, 1 insertion(+)
Git insists that we add files to the set we want to commit before actually committing anything. This allows us to commit our changes in stages and capture changes in logical portions rather than only large batches. For example, suppose we’re adding a few citations to relevant research to our thesis. We might want to commit those additions, and the corresponding bibliography entries, but not commit some of our work drafting the conclusion (which we haven’t finished yet).
To allow for this, Git has a special staging area where it keeps track of things that have been added to the current changeset but not yet committed.
The staging area is the middle ground between what you have done to your files (also known as the working directory) and what you had last committed (the HEAD commit). As the name implies, the staging area gives you space to prepare (stage) the changes that will be reflected on the next commit. (Coderefinery, 2023)
Staging Area
If you think of Git as taking snapshots of changes over the life of a
project, git add
specifies what will go in a
snapshot (putting things in the staging area), and
git commit
then actually takes the snapshot, and
makes a permanent record of it (as a commit). If you don’t have anything
staged when you type git commit
, Git will prompt you to use
git commit -a
or git commit --all
, which is
kind of like gathering everyone to take a group photo! However,
it’s almost always better to explicitly add things to the staging area,
because you might commit changes you forgot you made. (Going back to the
group photo simile, you might get an extra with incomplete makeup
walking on the stage for the picture because you used -a
!)
Try to stage things manually, or you might find yourself searching for
“git undo commit” more than you would like!
Checklist
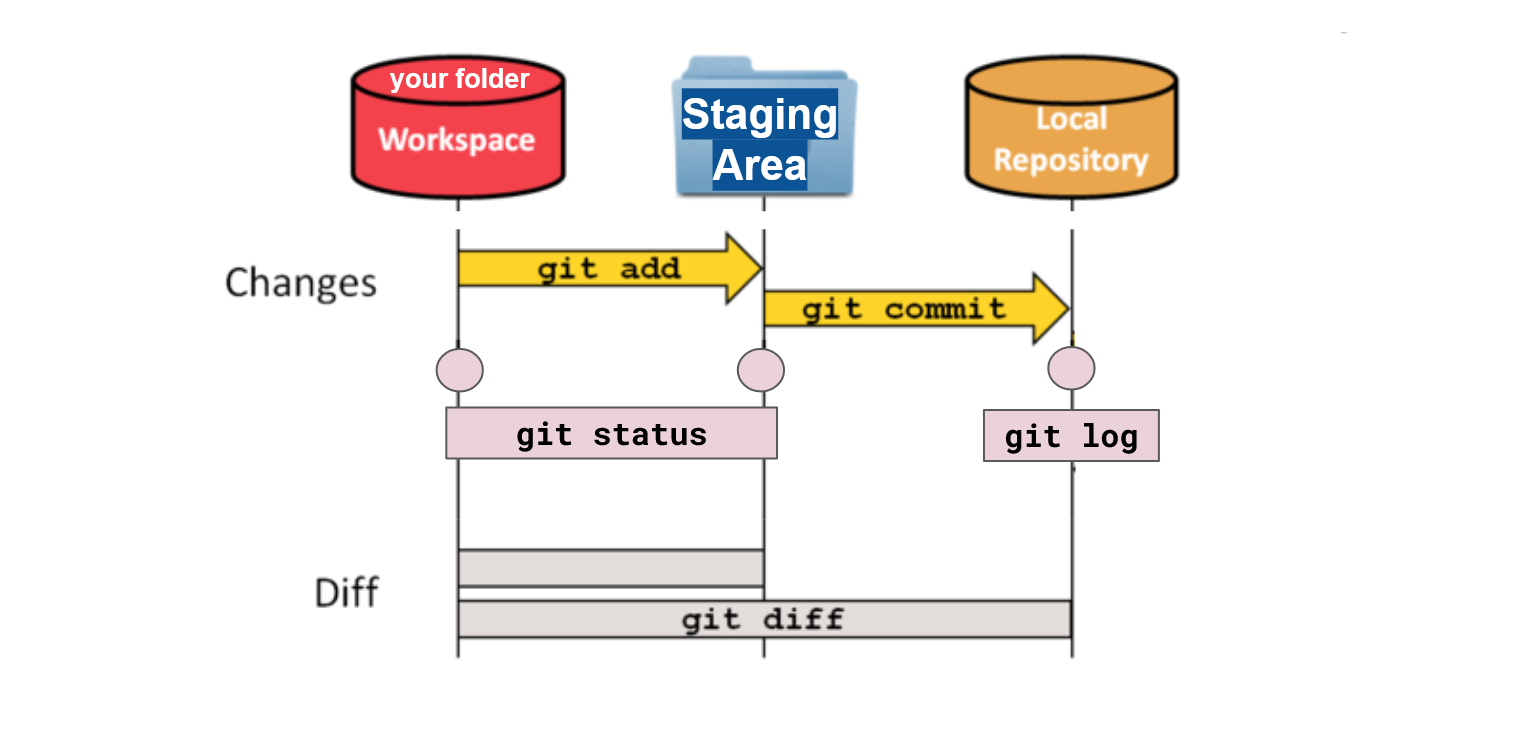
git status
to display the state
of the working directory and the staging area. git add
your
changes before you git commit
them to the Local repository.
Use the git log
to get the history of changes in it. Use
git diff
to compare these changes.Group Challenges
Choosing a Commit Message
Which of the following commit messages would be most appropriate for
the last commit made to sitrep.Rmd
?
- “Changes”
- “Added line ‘Maps illustrate the spread and impact of outbreak’ to sitrep.Rmd”
- “Discuss effects of Sitrep’ climate on the Mummy”
Answer 1 is not descriptive enough, and the purpose of the commit is unclear; and answer 2 is redundant to using “git diff” to see what changed in this commit; but answer 3 is good: short, descriptive, and imperative.
Committing Changes to Git
Which command(s) below would save the changes of
myfile.txt
to my local Git repository?
- Would only create a commit if files have already been staged.
- Would try to create a new repository.
- Is correct: first add the file to the staging area, then commit.
- Would try to commit a file “my recent changes” with the message myfile.txt.
Your turn!
Take 10 minutes to the following two sections:
- Practice the workflow
- Relevant callouts
If you want to keep practicing, move to the last one:
- Individual Challenges
Practice the workflow
Let’s watch as our changes to a file move from our editor to the staging area and into long-term storage. First, in the Console, we’ll add another line to the file:
R
usethis::edit_file("sitrep.Rmd")
OUTPUT
Comparison of attack rates in different age groups
This can identify priority groups for interventions
Maps illustrate the spread and impact of outbreak
Save the file.
In the Terminal, check the difference:
OUTPUT
diff --git a/sitrep.Rmd b/sitrep.Rmd
index 315bf3a..b36abfd 100644
--- a/sitrep.Rmd
+++ b/sitrep.Rmd
@@ -1,2 +1,3 @@
Comparison of attack rates in different age groups
This can identify priority groups for interventions
+Maps illustrate the spread and impact of outbreak
So far, so good: we’ve added one line to the end of the file (shown
with a +
in the first column). Now let’s put that change in
the staging area and see what git diff
reports:
There is no output: as far as Git can tell, there’s no difference between what it’s been asked to save permanently and what’s currently in the directory. However, if we do this:
OUTPUT
diff --git a/sitrep.Rmd b/sitrep.Rmd
index 315bf3a..b36abfd 100644
--- a/sitrep.Rmd
+++ b/sitrep.Rmd
@@ -1,2 +1,3 @@
Comparison of attack rates in different age groups
This can identify priority groups for interventions
+Maps illustrate the spread and impact of outbreak
it shows us the difference between the last committed change and what’s in the staging area. Let’s save our changes:
OUTPUT
[main 005937f] Add geospatial visualization objective
1 file changed, 1 insertion(+)
check our status:
OUTPUT
On branch main
nothing to commit, working tree clean
and look at the history of what we’ve done so far:
OUTPUT
commit 005937fbe2a98fb83f0ade869025dc2636b4dad5 (HEAD -> main)
Author: Vlad Dracula <vlad@tran.sylvan.ia>
Date: Thu Aug 22 10:14:07 2013 -0400
Add geospatial visualization objective
commit 34961b159c27df3b475cfe4415d94a6d1fcd064d
Author: Vlad Dracula <vlad@tran.sylvan.ia>
Date: Thu Aug 22 10:07:21 2013 -0400
Add purpose of attack rate analysis
commit f22b25e3233b4645dabd0d81e651fe074bd8e73b
Author: Vlad Dracula <vlad@tran.sylvan.ia>
Date: Thu Aug 22 09:51:46 2013 -0400
Start Sitrep with attack rate analysis
Relevant callouts
Word-based diffing
Sometimes, e.g. in the case of the text documents a line-wise diff is
too coarse. That is where the --color-words
option of
git diff
comes in very useful as it highlights the changed
words using colors.
Paging the Log
When the output of git log
is too long to fit in your
screen, git
uses a program to split it into pages of the
size of your screen. When this “pager” is called, you will notice that
the last line in your screen is a :
, instead of your usual
prompt.
- To get out of the pager, press Q.
- To move to the next page, press Spacebar.
- To search for
some_word
in all pages, press / and typesome_word
. Navigate through matches pressing N.
Limit Log Size
To avoid having git log
cover your entire terminal
screen, you can limit the number of commits that Git lists by using
-N
, where N
is the number of commits that you
want to view. For example, if you only want information from the last
commit you can use:
OUTPUT
commit 005937fbe2a98fb83f0ade869025dc2636b4dad5 (HEAD -> main)
Author: Vlad Dracula <vlad@tran.sylvan.ia>
Date: Thu Aug 22 10:14:07 2013 -0400
Add geospatial visualization objective
You can also reduce the quantity of information using the
--oneline
option:
OUTPUT
005937f (HEAD -> main) Add geospatial visualization objective
34961b1 Add purpose of attack rate analysis
f22b25e Start Sitrep with attack rate analysis
You can also combine the --oneline
option with others.
One useful combination adds --graph
to display the commit
history as a text-based graph and to indicate which commits are
associated with the current HEAD
, the current branch
main
, or other
Git references:
OUTPUT
* 005937f (HEAD -> main) Add geospatial visualization objective
* 34961b1 Add purpose of attack rate analysis
* f22b25e Start Sitrep with attack rate analysis
Directories
Two important facts you should know about directories in Git.
Git track files, not empty directories
- Git does not track directories on their own, only files within them. Try it for yourself using the Terminal:
Note, our newly created empty directory analyses
does
not appear in the list of untracked files even if we explicitly add it
(via git add
) to our repository. This is the
reason why you will sometimes see .gitkeep
files in
otherwise empty directories. Unlike .gitignore
, these files
are not special and their sole purpose is to populate a directory so
that Git adds it to the repository. In fact, you can name such files
anything you like.
- If you create a directory in your Git repository and populate it with files, you can add all files in the directory at once by:
Try it for yourself:
BASH
$ touch analyses/attack-rate.R analyses/geospatial.R
$ git status
$ git add analyses
$ git status
Before moving on, we will commit these changes.
To recap, when we want to add changes to our repository, we first
need to add the changed files to the staging area (git add
)
and then commit the staged changes to the repository
(git commit
):
Individual Challenges
Committing Multiple Files
The staging area can hold changes from any number of files that you want to commit as a single snapshot.
- Add some text to
sitrep.Rmd
noting your decision to consider a data cleaning preliminary step. - Create a new file
clean.Rmd
with your initial thoughts about Data as an step for you and your friends. - Add changes from both files to the staging area, and commit those changes.
The output below from sitrep.Rmd
reflects only content
added during this exercise. Your output may vary.
First we make our changes to the sitrep.Rmd
and
clean.Rmd
files:
R
usethis::edit_file("sitrep.Rmd")
OUTPUT
Maybe I should start with a data cleaning step.
R
usethis::edit_file("clean.Rmd")
OUTPUT
Data is a messy file and I definitely should consider a data cleaning step.
Save both files.
Now you can add both files to the staging area. We can do that in one line:
Or with multiple commands:
Now the files are ready to commit. You can check that using
git status
. If you are ready to commit use:
OUTPUT
[main cc127c2]
Write plans to start a data cleaning step
2 files changed, 2 insertions(+)
create mode 100644 clean.Rmd
bio
Repository
- Create a new Git repository on your computer called
bio
. - Write a three-line biography for yourself in a file called
me.md
, commit your changes - Modify one line, add a fourth line
- Display the differences between its updated state and its original state.
If needed, create a project out of the cases
folder:
R
usethis::create_project(path = "bio")
Initialise git:
R
usethis::use_git()
Create your biography file me.md
using the Rstudio
editor.
R
usethis::edit_file("me.md")
Save the file.
Once in place, in the Terminal add and commit it to the repository:
Modify the file as described (modify one line, add a fourth line). To
display the differences between its updated state and its original
state, use git diff
:
Key Points
-
git status
shows the status of a repository. - Files can be stored in a project’s working directory (which users see), the staging area (where the next commit is being built up) and the local repository (where commits are permanently recorded).
-
git add
puts files in the staging area. -
git commit
saves the staged content as a new commit in the local repository. - Write a commit message that accurately describes your changes.